After setting up your Android development environment, Developing an Android Application, and Created an Android Test environment it’s time to integrate your application and corresponding tests inside an automatic build environment. The steps to achieve this are:
- Create an maven project structure.
- Adding the specific Android setting to maven.
- Build and run the application.
- Build and run the tests.
This post isn’t about CI, it’s about how to setup an automatic build environment with use of Maven. I will use the Android application and corresponding tests which was developed inside my previous posts.
Create a Maven project structure
If you are brand new to it you may wish to first check out Maven in 5 minutes. Basically create a parent-child structure as shown below.
parent
|— Android
| |— pom.xml
|— AndroidTest
| |— pom.xml
|— pom.xml
In this post I’m using maven version 2.2.1.
Adding the specific Android setting to maven
I will be using the maven-android-plugin to build the Android application and Robotium to test the application. In this post I’m using maven-android-plugin version 2.5.2.
The parent project pom.xml must reference the maven-android-plugin, along with the android API level targeted, here I cite version 2.2 (api level 8 )
<groupId>com.jayway.maven.plugins.android.generation2</groupId>
<artifactId>maven-android-plugin</artifactId>
<configuration>
<sdk>
<platform>2.2</platform>
</sdk>
<emulator>
<avd>Device</avd>
<wait>2000</wait>
</emulator>
<deleteConflictingFiles>true</deleteConflictingFiles>
<undeployBeforeDeploy>true</undeployBeforeDeploy>
</configuration>
<extensions>true</extensions>
</plugin>
Build and run the application
- To build the Android.apk, we need add a version of the android platform as a build path dependency in the Android project pom.xml file.
- Add the goal into the Android project pom.xml to deploy the apk onto an Android emulator.
- Specify the source directory.
- Run the emulator
- Run the maven command mvn clean install inside the Android project.
- You should get the following result.
<dependency>
<groupId>com.google.android</groupId>
<artifactId>android</artifactId>
<scope>provided</scope>
</dependency>
</dependencies>
<plugin>
<groupId>com.jayway.maven.plugins.android.generation2</groupId>
<artifactId>maven-android-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>deploy</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
</plugin>
</plugins>
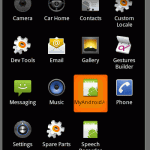
Build and run the tests
- To run the tests inside the AndroidTest project, we need add the following dependency inside the AndroidTest project pom.xml file.
- Add the source code of the Android application which it tests.
- Specify the source directory.
- Run the emulator
- Run the maven command mvn clean install inside the parent project.
- You should get the following result.
<groupId>com.jayway.android.robotium</groupId>
<artifactId>robotium-solo</artifactId>
<version>1.4.0</version>
</dependency>
<dependency>
<groupId>com.google.android</groupId>
<artifactId>android</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>com.google.android</groupId>
<artifactId>android-test</artifactId>
<scope>provided</scope>
</dependency>
<groupId>com.my.android</groupId>
<artifactId>Android</artifactId>
<version>${project.version}</version>
<scope>compile</scope>
<type>jar</type>
</dependency>

Here’s the fully-configured project pom.xml files using ALL of the above:
parent
|— Android
| |— pom.xml
|— AndroidTest
| |— pom.xml
|— pom.xml